C++ Exercises: Compute square root of a given non-negative integer
C++ Math: Exercise-23 with Solution
Write a C++ program to compute square root of a given non-negative integer. Return type should be an integer.
Sample Input: n = 81
Sample Output: Square root of 81 = 9
Sample Input: n = 8
Sample Output: Square root of 8 = 2
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
int square_root(int num1) {
long left_part = 0;
long right_part = num1 / 2 + 1;
while (left_part <= right_part) {
long mid = left_part + (right_part - left_part) / 2;
long result = mid * mid;
if (result == (long) num1) {
return (int) mid;
} else if (result > num1) {
right_part = mid - 1;
} else {
left_part = mid + 1;
}
}
return (int) right_part;
}
int main() {
int n = 81;
cout << "\nSquare root of " << n << " = " << square_root(n) << endl;
n = 8;
cout << "\nSquare root of " << n << " = " << square_root(n) << endl;
n = 627;
cout << "\nSquare root of " << n << " = " << square_root(n) << endl;
n =225;
cout << "\nSquare root of " << n << " = " << square_root(n) << endl;
return 0;
}
Sample Output:
Square root of 81 = 9 Square root of 8 = 2 Square root of 627 = 25 Square root of 225 = 15
Flowchart:
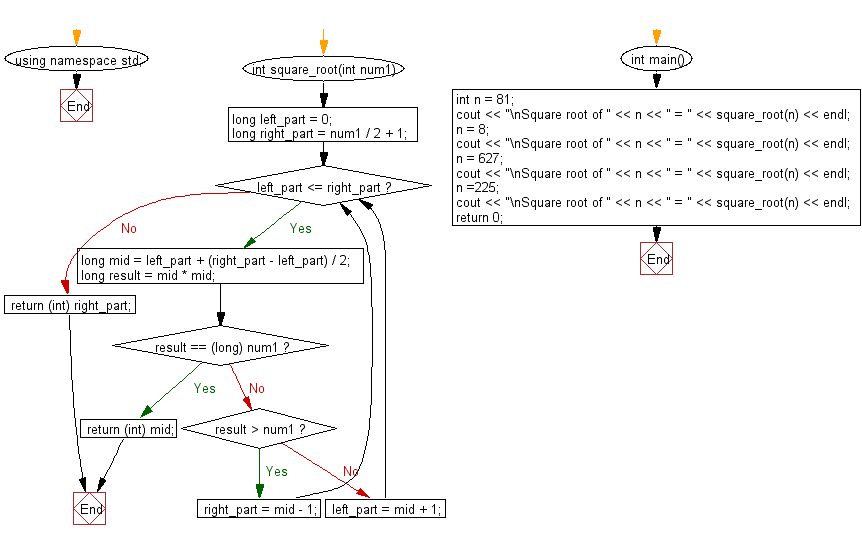
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to compute the sum of two given binary strings.
Next: Write a C++ program to count the prime numbers less than a given positive number.
What is the difficulty level of this exercise?