C++ Exercises: Multiply two integers without using multiplication, division, bitwise operators, and loops
C++ Math: Exercise-17 with Solution
Write a C++ program to multiply two integers without using multiplication, division, bitwise operators, and loops.
Sample Input: 8, 9
Sample Output: 72
Sample Input: -11, 19
Sample Output: -209
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
int multiply_two_nums(int x, int y)
{
if(y == 0)
return 0;
if(y > 0 )
return (x + multiply_two_nums(x, y-1 ));
if(y < 0 )
return - multiply_two_nums(x, -y);
}
int main()
{
cout << multiply_two_nums(8, 9) << endl;
cout << multiply_two_nums(-11, 19) << endl ;
return 0;
}
Sample Output:
72 -209
Flowchart:
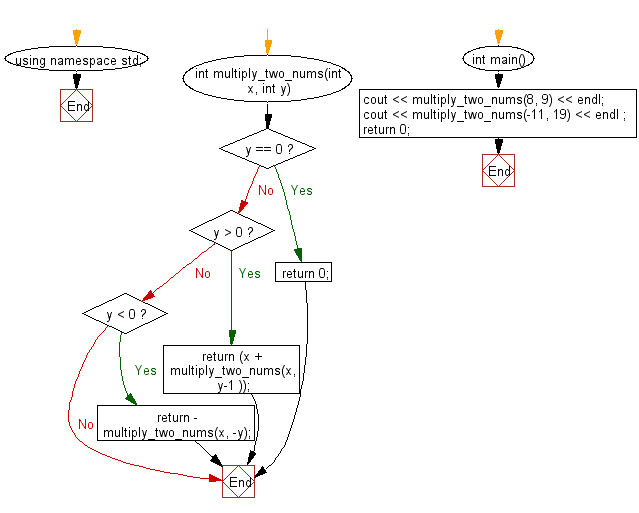
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ programming to find the nth digit of number 1 to n.
Next: Write a C++ program to convert a given integer to a roman numeral.
What is the difficulty level of this exercise?