C++ Exercises: Convert a given number into hours and minutes
C++ Basic: Exercise-83 with Solution
Write a C++ program to convert a given number into hours and minutes. Separate the number of hours and minutes with a colon.
For example if a given number is 67 the output should be 1:7
Pictorial Presentation:
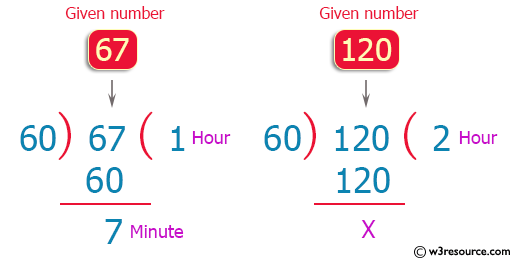
Sample Solution:
C++ Code :
#include <iostream>
#include <string>
using namespace std;
void Time_Convert(int num) {
bool flag;
int hr = 0;
do
{
flag = false;
if (num >= 60)
{
hr++;
num -= 60;
flag = true;
}
} while (flag);
cout << "\nH:M " << hr << ":" << num << endl;
}
int main() {
Time_Convert(67);
Time_Convert(60);
Time_Convert(120);
Time_Convert(40);
return 0;
}
Sample Output:
H:M 1:7 H:M 1:0
Flowchart:
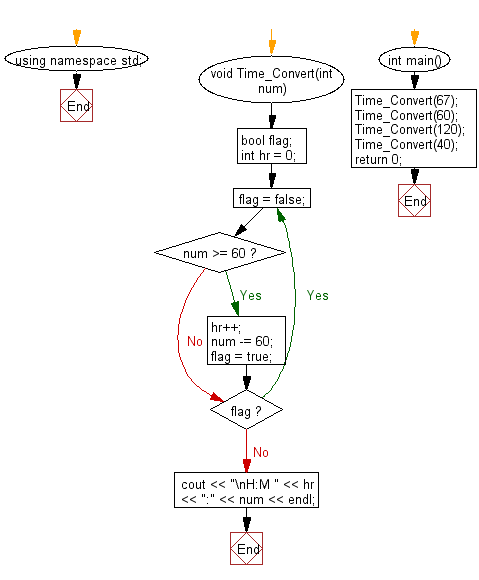
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program which reads a list of pairs of a word and a page number, and prints the word and a list of the corresponding page numbers.
Next: Write a C++ program to check whether the sequence of the numbers in a given array is a “Arithmetic” or “Geometric” sequence.
What is the difficulty level of this exercise?