C++ Exercises: Add all the numbers from 1 to a given number
C++ Basic: Exercise-66 with Solution
Pictorial Presentation:
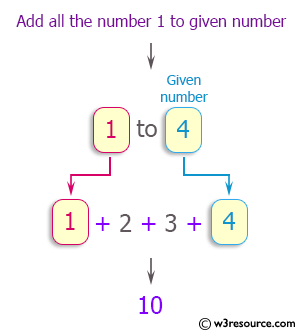
Write a C++ program to add all the numbers from 1 to a given number.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
int Add_1_to_given_number(int n) {
int total = 0;
for (int x = 1; x <= n; x++)
{
total += x;
}
return total;
}
int main() {
cout << "\nAdd 1 to 4: " << Add_1_to_given_number(4);
cout << "\nAdd 1 to 100: " << Add_1_to_given_number(100);
return 0;
}
Sample Output:
Add 1 to 4: 10 Add 1 to 100: 5050
Flowchart:
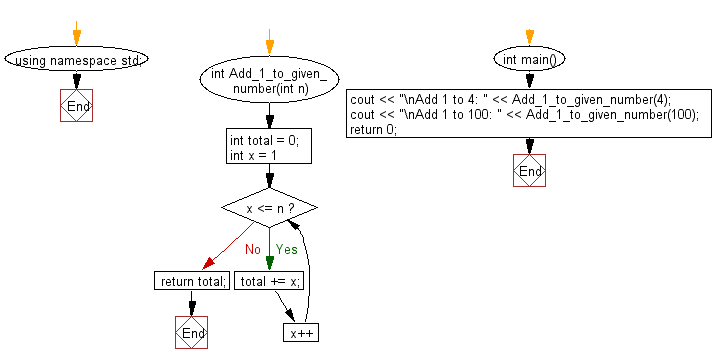
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check whether given length of three side form a right triangle.
Next: Write a C++ program to which prints the central coordinate and the radius of a circumscribed circle of a triangle which is created by three points on the plane surface.
What is the difficulty level of this exercise?