C Exercises: Delete node from the last of a doubly linked list
C Linked List : Exercise-18 with Solution
Write a program in C to delete a node from the last of a doubly linked list.
Pictorial Presentation:
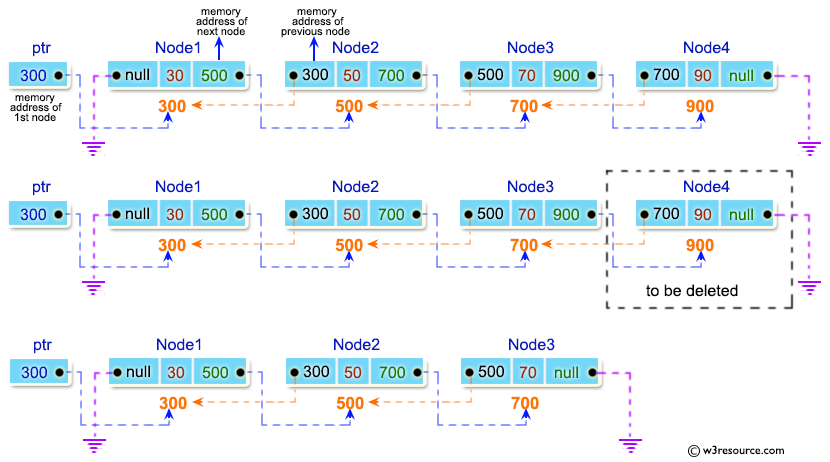
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
struct node {
int num;
struct node * preptr;
struct node * nextptr;
}*stnode, *ennode;
void DlListcreation(int n);
void DlListDeleteLastNode();
void displayDlList(int a);
int main()
{
int n,num1,a,insPlc;
stnode = NULL;
ennode = NULL;
printf("\n\n Doubly Linked List : Delete node from the last of a doubly linked list :\n");
printf("-----------------------------------------------------------------------------\n");
printf(" Input the number of nodes (3 or more ): ");
scanf("%d", &n);
DlListcreation(n);
a=1;
displayDlList(a);
DlListDeleteLastNode();
a=2;
displayDlList(a);
return 0;
}
void DlListcreation(int n)
{
int i, num;
struct node *fnNode;
if(n >= 1)
{
stnode = (struct node *)malloc(sizeof(struct node));
if(stnode != NULL)
{
printf(" Input data for node 1 : "); // assigning data in the first node
scanf("%d", &num);
stnode->num = num;
stnode->preptr = NULL;
stnode->nextptr = NULL;
ennode = stnode;
for(i=2; i<=n; i++)
{
fnNode = (struct node *)malloc(sizeof(struct node));
if(fnNode != NULL)
{
printf(" Input data for node %d : ", i);
scanf("%d", &num);
fnNode->num = num;
fnNode->preptr = ennode; // new node is linking with the previous node
fnNode->nextptr = NULL; // set next address of fnnode is NULL
ennode->nextptr = fnNode; // previous node is linking with the new node
ennode = fnNode; // assign new node as last node
}
else
{
printf(" Memory can not be allocated.");
break;
}
}
}
else
{
printf(" Memory can not be allocated.");
}
}
}
void DlListDeleteLastNode()
{
struct node * NodeToDel;
if(ennode == NULL)
{
printf(" Delete is not possible. No data in the list.\n");
}
else
{
NodeToDel = ennode;
ennode = ennode->preptr; // move the previous address of the last node to 2nd last node
ennode->nextptr = NULL; // set the next address of last node to NULL
free(NodeToDel); // delete the last node
}
}
void displayDlList(int m)
{
struct node * tmp;
int n = 1;
if(stnode == NULL)
{
printf(" No data found in the List yet.");
}
else
{
tmp = stnode;
if (m==1)
{
printf("\n Data entered in the list are :\n");
}
else
{
printf("\n After deletion the new list are :\n");
}
while(tmp != NULL)
{
printf(" node %d : %d\n", n, tmp->num);
n++;
tmp = tmp->nextptr; // current pointer moves to the next node
}
}
}
Sample Output:
Doubly Linked List : Delete node from the last of a doubly linked list : ----------------------------------------------------------------------------- Input the number of nodes (3 or more ): 3 Input data for node 1 : 1 Input data for node 2 : 2 Input data for node 3 : 3 Data entered in the list are : node 1 : 1 node 2 : 2 node 3 : 3 After deletion the new list are : node 1 : 1 node 2 : 2
Flowchart:
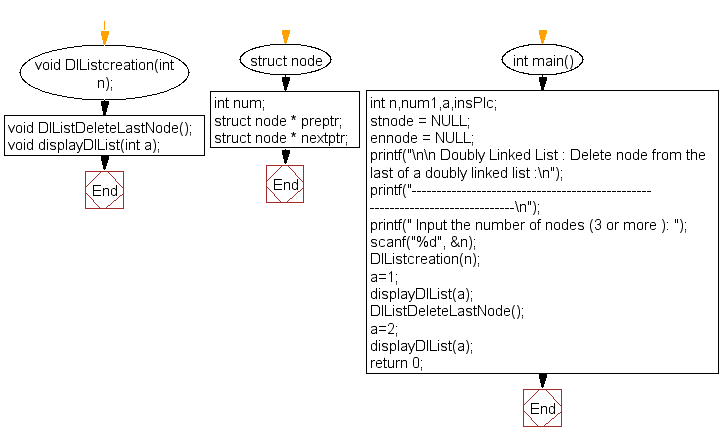
DlListcreation() :
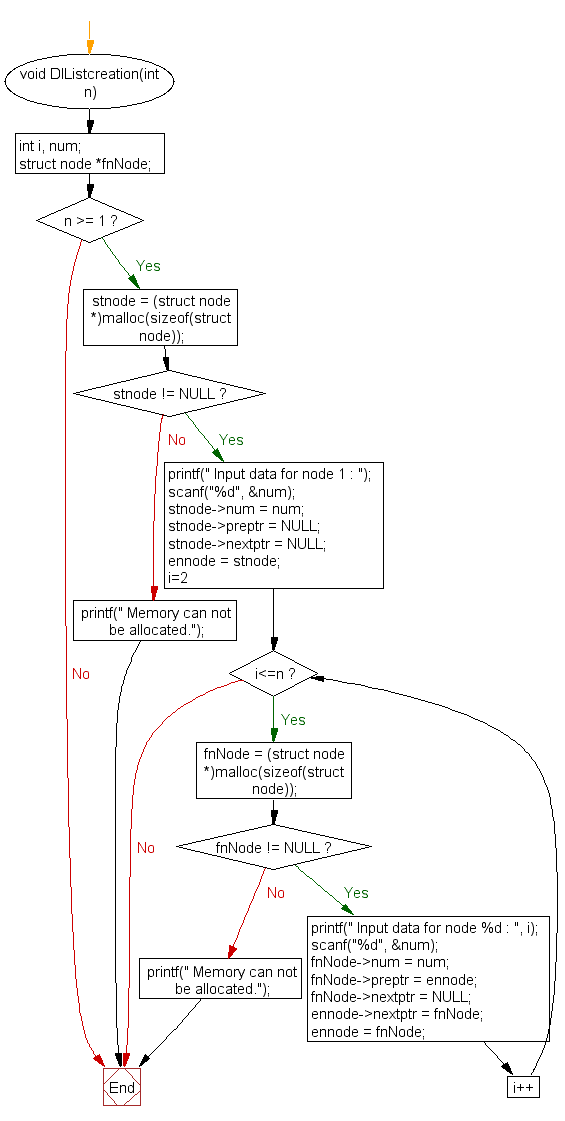
DlListDeleteLastNode() :
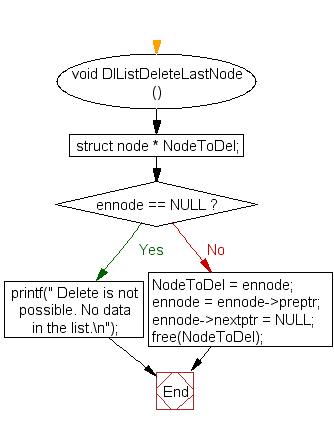
displayDlList() :
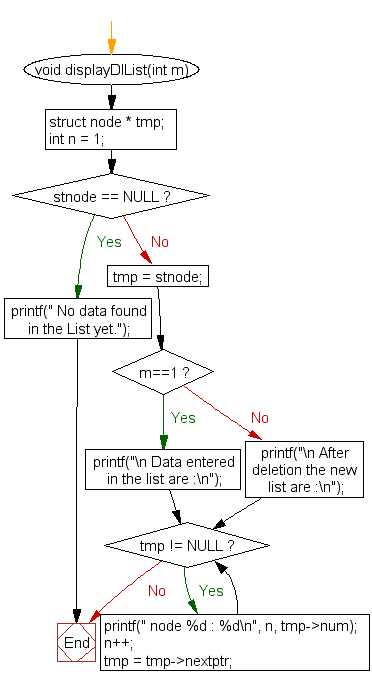
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to delete a node from the beginning of a doubly linked list.
Next: Write a program in C to delete a node from any position of a doubly linked list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
C Programming: Tips of the Day
Static variable inside of a function in C
The scope of variable is where the variable name can be seen. Here, x is visible only inside function foo().
The lifetime of a variable is the period over which it exists. If x were defined without the keyword static, the lifetime would be from the entry into foo() to the return from foo(); so it would be re-initialized to 5 on every call.
The keyword static acts to extend the lifetime of a variable to the lifetime of the programme; e.g. initialization occurs once and once only and then the variable retains its value - whatever it has come to be - over all future calls to foo().
Ref : https://bit.ly/3fOq7XP
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework