Introduction to modules
Angular applications are modular by default and Angular was built with its own modularity system called NgModules.
NgModules are containers for a cohesive block of code dedicated to an application domain, a workflow, or a closely related set of capabilities. They can contain components, service providers, and other code files whose scope is defined by the containing NgModule. They can import functionality that is exported from other NgModules, and export selected functionality for use by other NgModules.
Every Angular app has at least one NgModule class, the root module, which is conventionally named AppModule and resides in a file named `app.module.ts`. Your Angular application is launched by bootstrapping the root NgModule.
NgModule metadata
An NgModule is defined by a class decorated with @NgModule(). The @NgModule() decorator is a function that takes a single metadata object, whose properties describe the module. The most important properties are as follows.
- declarations: The components, directives, and pipes that belong to this NgModule.
- exports: The subset of declarations that should be visible and usable in the component templates of other NgModules.
- imports: Other modules whose exported classes are needed by component templates declared in this NgModule.
- providers: Creators of services that this NgModule contributes to the global collection of services; they become accessible in all parts of the app. (You can also specify providers at the component level, which is often preferred.)
- bootstrap: The main application view, called the root component, which hosts all other app views. Only the root NgModule should set the bootstrap property.
Below is a code snippet illustrating a simple root NgModule definition.
TypeScript Code:
src/app/app.module.ts;
content_copyimport { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
@NgModule({
imports: [ BrowserModule ],
providers: [ Logger ],
declarations: [ AppComponent ],
exports: [ AppComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule {
}
Live Demo:
It is just a code snippet explaining a particular concept and may not have any output
See the Pen A simple root NgModule definition. by w3resource (@w3resource) on CodePen.
In the above code snippet illustration, AppComponent is included in the exports list just for illustration; it isn't actually necessary in this example. This is because a root NgModule has no reason to export anything because other modules don't need to import the root NgModule.
NgModules and components
NgModules spins up the compilation of its components. A root NgModule always has a root component that is created during bootstrap, during development, any NgModule can contain any number of components depending on your design.
These components can be loaded through the router or created through the template. The components that belong to an NgModule share a compilation context.
The diagram below, illustrates the relationship between components and NgModules.
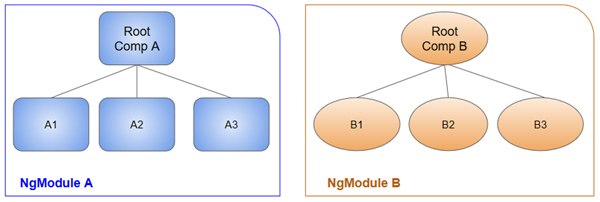
Views are defined by a component and its template. A component can contain a view hierarchy, which allows you to define arbitrarily complex areas of the screen that can be created, modified, and destroyed as a unit. A view hierarchy can mix views defined in components that belong to different NgModules. This combination of components in the view hierarchy, is illustrated with
the diagram below:
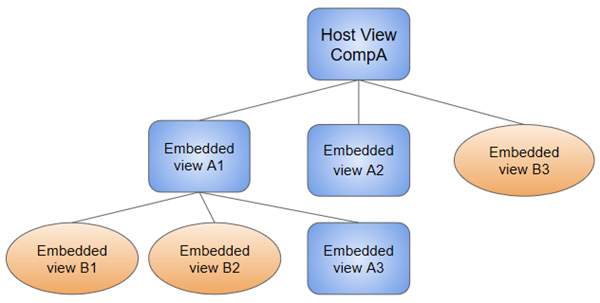
When components are created, it's associated directly with a single view, called the host view. The host view can be the root of a view hierarchy, which can contain embedded views, which can in turn be the host views of other components. These components can be in the same NgModule, or can be imported from other NgModules.
In the nesting of views, the nesting tree can be nested to any depth, depending on what you want to achieve.
NgModules and JavaScript modules
When NgModule and JavaScript (ES2015) modules are compared side by side we discovered that the NgModule system is different from and unrelated to the JavaScript (ES2015) module system for managing collections of JavaScript objects.
These are complementary module systems that you can use together to write your apps. In JavaScript each file is a module and all objects defined in the file belong to that module. The module declares some objects to be public by marking them with the export keyword. Other JavaScript modules use import statements to access public objects from other modules. Examples of JavaScript import and export are illustrated in the example below.
TypeScript Code:
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
export class AppModule {
}
Live Demo:
It is just a code snippet explaining a particular concept and may not have any output
See the Pen Example of JavaScript import and export by w3resource (@w3resource) on CodePen.
Angular libraries
Angular uses a collection of JavaScript modules. You can think of them as libraries. Each Angular library name begins with the @angular prefix. The Libraries are installed using the Node Package Manager (NPM) and imported using the JavaScript import statement.
For example, to import Angular Component decorator from the @angular/core library we say:
`import {Component} from '@angular/core';`
You also import NgModules from Angular libraries using JavaScript import statements. For example, the following code imports the BrowserModule NgModule from the platform-browser library.
`import {BrowserModule} from '@angular/platform-browser';`
In order to access the data from the imported modules, we need to add the imported libraries to the @NgModule metadata imports like this, as shown below:
TypeScript Code:
@NgModule({
imports:[ BrowserModule ],
})
Live Demo:
It is just a code snippet explaining a particular concept and may not have any output
See the Pen Adding an imported library to @NgModule Metadata by w3resource (@w3resource) on CodePen.
In this way you're using the Angular and JavaScript module systems together. Although it's easy to confuse the two systems, which share the common vocabulary of "imports" and "exports", you will become familiar with the different contexts in which they are used.
Previous: User Input
Next:
Template Syntax
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework